By now everyone has heard about Machine Learning and how it will change everything. But very few have an idea how to start to change everything. I hope that when you try the steps in this blog, or even read about it will help you understand how to get started to change things with your PLCnext Controller. In this blog I go about training your first ML model, converting it to the ONNX standard and inferencing the model on a PLCnext controller. As to not go and make things to overwhelming I’ll be using the famous Iris dataset to build our model.
Before we start, it should be very clear what we’ll try to achieve. Hence I will give a small explanation about the topics handled in this blog. My references can be found at the end of this blog.
Introduction to Machine Learning
What is machine Learning
So, I guess we should start with explaining what machine learning is. The essence of machine learning is that we’ll try to find patterns in datasets with the use of statistics and algorithms. We differentiate between three mayor types of machine learning: supervised machine learning, unsupervised machine learning and reinforcement learning. Supervised learning is the most used “flavor” today and we’ll be using supervised learning in this blog. In supervised learning we label data en tell the machine exactly what patterns we are looking for.
In unsupervised learning we don’t label our data and we let the machine go find it own patterns, as this technique has less obvious applications, unsupervised learning is less popular.
Lastly, in reinforcement learning an algorithm learns by trial and error to achieve a stated objective. It just tries a lot of things and gets a reward or penalized depending if it was a good or a bad action. Googles AlphaGo is a famous example of reinforcement learning.
The Iris flower data set
According to Wikipedia the Iris flower dataset is:
The Iris flower data set or Fisher’s Iris data set is a multivariate data set introduced by the British statistician, eugenicist, and biologist Ronald Fisher in his 1936 paper The use of multiple measurements in taxonomic problems as an example of linear discriminant analysis.[1] It is sometimes called Anderson’s Iris data set because Edgar Anderson collected the data to quantify the morphologic variation of Iris flowers of three related species.[2]
Ok, fine but how does it look?
In the Iris dataset there are 5 fields: sepal length, sepal width, petal length, petal width and the variety of Iris flower. Our goal today is to find the type of Iris flower when we know the sepal length, sepal width, petal length and petal width. So we’ll train the model to classify the type of flower. As you could have guessed this type of machine learning is classification.
Machine learning can also be used to predict a value in the data set. This is procedure is named regression and uses different algorithms than classification.
The Algorithm
An algorithm is the list of instructions and rules that a computer needs to do to complete a task.
Today we’ll be using the “Decision Tree Classifier” not because it is perfect for the task, but it is very intuitive and can be easily understood without fancy mathematics. Here you can find an example of an decision tree for our Iris flower data set.
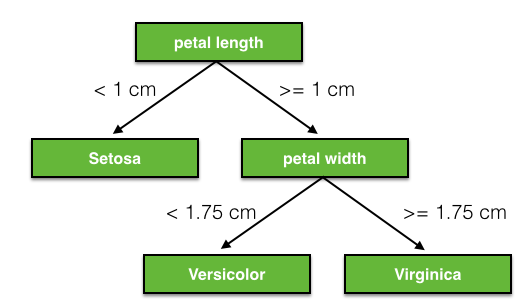
ONNX
As you can imagine Machine Learning models can come in a wide variety of formats and need to run on a lot of different hardware with different acceleration methods. The Open Neural Network Exchange tries to mitigate that problem. It gets used in open office, azure and countless other applications around the world. It gets almost certainly used on the device you’re reading this blog.
ONNX is an open format built to represent machine learning models. ONNX defines a common set of operators – the building blocks of machine learning and deep learning models – and a common file format to enable AI developers to use models with a variety of frameworks, tools, runtimes, and compilers.
To run onnx models we need the onnx runtime, this comes with it challenges. Especially on arm processors, but with the docker images provided, you should be fine!
Technical walktrough
Prerequisites
I’m using an AXC F 2152 controller with firmware 2021.0 LTS installed and an Ubuntu 20.04 VM to train the model. The scripts for training and inferencing the model are provided but the setup of the Ubuntu VM is out of scope of the blog. You can find good explanations on how to install the needed Python packages and all used Packages should install correctly with pip3.
On the PLCnext controller we’ll need a container engine installed.You can find a good explanation of the procedure here.
A similar procedure with the AXC F 3152 is also possible.
You’ll need at least a minimal experience with Python and containers for this blog.
Training the model
Download the contents of this GitHub repository and make sure all necessary packages are installed.
The first script we are going to run is the train script were we are going to fit our model to the iris data set.
Below you can find a code snipped from this training script. This script will create a “.onnx” file witch contains are trained model.
# Slit the dataset in a training and testing dataset
iris = load_iris()
X, y = iris.data, iris.target
X_train, X_test, y_train, Y_test = train_test_split(X, y)
# Define the model and fit the model with training data and print information about the model
clr = DecisionTreeClassifier()
clr.fit(X_train, y_train)
print(clr)
#Convert the model from sklearn format to ONNX (Open Neural Network Exchange)
initial_type = [('float_input', FloatTensorType([None, 4]))]
onx = convert_sklearn(clr, initial_types=initial_type)
with open("decision_tree_iris.onnx", "wb") as f:
f.write(onx.SerializeToString())
Inferencing on Ubuntu
Check your model by running it on your developing machine. When you run the inferencing script you should get 2 integers corresponding to a type of Iris flower.
import numpy as np
import onnxruntime as rt
X_test = np.array([[5.8,4.0,1.2,0.2],[7.7,3.8,6.7,2.2,]])
sess = rt.InferenceSession("decision_tree_iris.onnx")
input_name = sess.get_inputs()[0].name
label_name = sess.get_outputs()[0].name
pred_onx = sess.run(
[label_name], {input_name: X_test.astype(np.float32)})[0]
print(pred_onx)
output : [0 2]
Inferencing on PLCnext
Open your favorite sFTP client and drop the “.onnx” and “inference.py” repository in /opt/plcnext/onnx on your PLCnext controller. Proceed with executing the next command as root:
balena-engine run -it --name onnx -v /opt/plcnext/onnx/:/app pxcbe/onnx-runtime-arm32v7
run the python inference script with
cd /app
python3 /app/inference.py
If all went well, you get the same output as with the inference on your Ubuntu VM! Congratualtions, you’ve made it till the end. Now go change things!
How to implement in an application?
Actually, we are not done yet. I mean, classifying iris flowers is fun but I can’t imagine multiple applications for it on a logical controller. You’ll need to come up with your own model and create an API for that model so you can inference with it. You can choose to deliver data to the model with OPC UA or build a custom REST-endpoint for it. Anyhow you’ll need to write some more code than provided by me.
Taking into account that building the images literately took me days and a sleepless night, I suggest you build your image on top of the image provided. In the reference you can find a good resource for building a Python container app.
References:
https://github.com/PLCnext/Docker_GettingStarted
https://www.wintellect.com/containerize-python-app-5-minutes/
Leave a Reply
You must be logged in to post a comment.